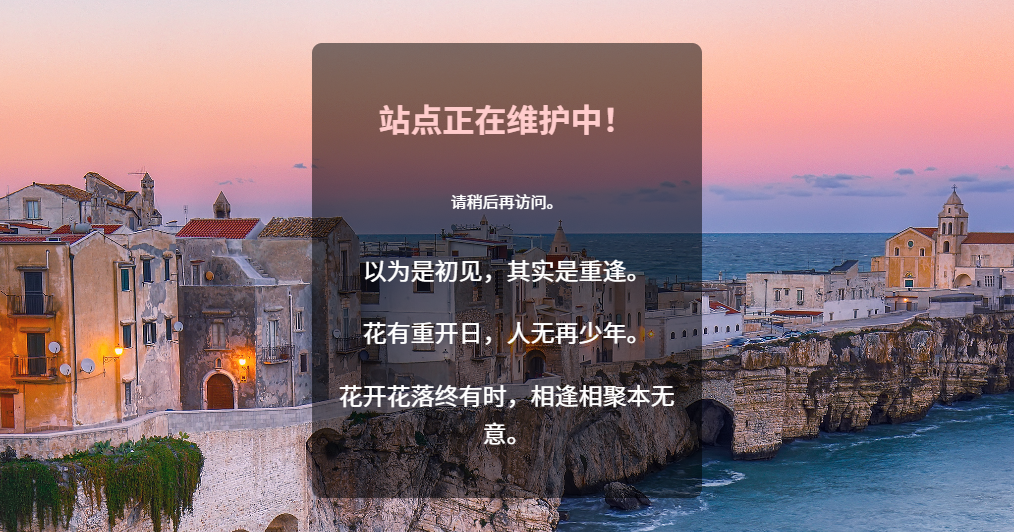
网站暂停访问时的显示样式html模板
AI-摘要
Fsx GPT
AI初始化中...
介绍自己
生成本文简介
推荐相关文章
前往主页
前往tianli博客
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>暂停访问</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
text-align: center;
background-image: url('https://p.muxin.aifsx.cn/2024/07/19/669a58b736cde.jpg');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
background-attachment: fixed;
height: 100vh;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
color: white;
}
.message {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
background-color: rgba(0, 0, 0, 0.5);
padding: 20px;
border-radius: 10px;
text-align: center;
width: 350px; /* 设置固定的宽度 */
min-height: 400px; /* 设置最小高度 */
margin: auto; /* 自动居中 */
}
.maintenance {
font-size: 32px;
font-weight: bold;
color: #ffcccc;
}
#content {
font-size: 24px;
color: #fff;
line-height: 1.6;
visibility: hidden; /* 先隐藏文字 */
}
footer {
margin-top: auto; /* 将footer推到页面底部 */
padding: 20px;
font-size: 16px;
color: #ffffff;
background-color: rgba(0, 0, 0, 0.5);
width: 100%;
}
footer a {
color: blue; /* 设置链接颜色为蓝色 */
text-decoration: none; /* 去掉下划线 */
}
footer a:hover {
color: purple; /* 鼠标悬停时变为紫色 */
text-decoration: underline; /* 悬停时添加下划线 */
}
</style>
</head>
<body>
<div class="message">
<p class="maintenance">站点正在维护中!</p>
<p>请稍后再访问。</p>
<div id="content"></div>
</div>
<footer>
<p>© 2024-2026 <a href="https://xn--otsr53afot.com/" target="_blank">“老朝奉”</a> & <a href="https://cloud.aifsx.cn/" target="_blank">“月落”</a> All Rights Reserved.</p>
</footer>
<script>
const paragraphs = [
"以为是初见,其实是重逢。\n",
"花有重开日,人无再少年。\n",
"花开花落终有时,相逢相聚本无意。\n"
];
let i = 0;
let j = 0;
let currentParagraph = "";
const contentDiv = document.getElementById('content');
// 预先渲染所有内容
contentDiv.textContent = paragraphs.join(""); // 预渲染所有文字
contentDiv.style.visibility = "visible"; // 设为可见,但内容将逐字显示
contentDiv.textContent = ""; // 清空内容,准备逐字输出
function displayNextChar() {
if (i < paragraphs.length) {
currentParagraph = paragraphs[i]; // 获取当前段落
if (j < currentParagraph.length) {
// 如果遇到换行符,创建一个新的 <p> 标签
if (currentParagraph[j] === '\n') {
const newParagraph = document.createElement('p');
contentDiv.appendChild(newParagraph);
} else {
// 将字符逐个添加到页面上
const lastParagraph = contentDiv.lastElementChild || document.createElement('p');
lastParagraph.textContent += currentParagraph[j];
if (!contentDiv.contains(lastParagraph)) {
contentDiv.appendChild(lastParagraph);
}
}
j++;
setTimeout(displayNextChar, 150); // 每150毫秒输出一个字
} else {
// 当一段文字结束后,进入下一段
i++;
j = 0;
setTimeout(displayNextChar, 500); // 新段落的延迟时间稍微长一点
}
}
}
displayNextChar();
</script>
</body>
</html>
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 老朝奉
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果